网页上的视频播放器是如何编写的?
作者:卡卷网发布时间:2025-01-19 22:33浏览数量:180次评论数量:0次
前几天需要在程序中使用视频播放功能,之后查找了一下资料,发现原来还有video.js这么强大的视频播放器,今天这篇文章就来总结下video.js的用法。
一、获取和引入 Video.js
- 通过 CDN 获取 Video.js:
- 可以从 jsdelivr 或 bootcdn 获取 Video.js 的 CDN 链接。以下是以 jsdelivr 为例的 CDN 链接:
<link href="https://unpkg.com/video.js@7.10.2/dist/video-js.min.css" rel="stylesheet">
<script src="https://unpkg.com/video.js@7.10.2/dist/video.min.js"></script>
- 引入 Video.js:
- 创建一个
<video>
标签,并包含data-setup
属性。data-setup
属性可以包含 Video.js 的各种配置。如果不需要配置,可以传一个空对象 `{}```:
<video
id="my-player"
class="video-js"
controls
preload="auto"
width="640"
height="264"
poster="http://vjs.zencdn.net/v/oceans.png"
data-setup="{}"
>
<source src="http://vjs.zencdn.net/v/oceans.mp4" type="video/mp4" />
<p class="vjs-no-js">
如果想使用 Video.js,请确保浏览器可以运行 JavaScript,并且支持
<a href="https://videojs.com/html5-video-support/" target="_blank">HTML5 video</a>
</p>
</video>
二、配置和定制
- 配置选项:
- Video.js 提供了多种配置选项,允许你定制播放器的行为和外观。常见的配置选项包括
autoplay
、loop
、muted
等:
var player = videojs('my-video', {
autoplay: true,
loop: true,
muted: true
});
- 定制播放器外观:
- 通过 CSS 定制播放器的外观:
.video-js .vjs-control-bar {
background: #000;
}
三、插件和扩展
- 使用插件:
- Video.js 拥有丰富的插件生态系统,允许你轻松添加额外的功能。例如,使用
videojs-contrib-hls
插件来播放 HLS 格式的视频:
npm install videojs-contrib-hls
import 'videojs-contrib-hls';
var player = videojs('my-video');
- 自定义插件:
- 你可以编写自己的插件来扩展 Video.js 的功能:
videojs.register
Plugin('myPlugin', function() {
var player = this;
player.on('play', function() {
console.log('Video is playing!');
});
});
var player = videojs('my-video');
player.myPlugin();
四、事件处理和 API
- 事件处理:
- Video.js 提供了丰富的事件机制,你可以监听播放器的各种事件,如播放、暂停、结束等:
var player = videojs('my-video');
player.on('play', function() {
console.log('Video is playing!');
});
player.on('pause', function() {
console.log('Video is paused!');
});
player.on('ended', function() {
console.log('Video has ended!');
});
- 使用 API:
- Video.js 提供了多种 API 方法,允许你控制播放器的行为:
var player = videojs('my-video');
// 播放视频
player.play();
// 暂停视频
player.pause();
// 设置当前播放时间为 10 秒
player.currentTime(10);
五、响应式设计和跨浏览器支持
- 响应式设计:
- Video.js 支持响应式设计,允许你创建适应不同屏幕尺寸的播放器:
.video-js {
width: 100%;
height: auto;
}
- 你还可以使用 Video.js 提供的
fluid
选项,使播放器根据容器大小自适应:
var player = videojs('my-video', {
fluid: true
});
- 跨浏览器支持:
- Video.js 兼容大多数现代浏览器,并提供了回退机制以支持旧版浏览器:
var player = videojs('my-video', {
techOrder: ['html5', 'flash']
});
六、项目管理和协作
在开发和维护使用 Video.js 的项目时,项目管理和协作工具可以极大地提高效率和团队协作能力。推荐使用以下两个系统:
- Vue.js 集成示例:
- 以下是一个 Vue.js 组件示例,展示了如何在 Vue.js 项目中使用 Video.js:
<template>
<video
ref="video"
controls
class="video-js vjs-default-skin vjs-big-play-centered"
width="600"
height="400"
>
<source :src="src" />
</video>
</template>
<script>
export default {
props: ["volume", "src"],
data() {
return {
player: null,
volumeVideo: this.volume,
};
},
methods: {
play() {
this.player.src({ src: this.src });
this.player.load(this.src);
this.player.play(this.volumeVideo);
},
stop() {
this.player.pause();
},
reload() {
this.stop();
this.player.load({});
this.play();
},
forward() {
const currentTime = this.player.currentTime();
this.player.currentTime(currentTime + 5);
},
back() {
const currentTime = this.player.currentTime();
this.player.currentTime(currentTime - 5);
},
volumeUp() {
this.player.volume(this.volumeVideo + 0.1);
},
volumeDown() {
this.player.volume(this.volumeVideo - 0.1);
},
toggleTv(obj) {
this.player.src(obj.src);
this.player.load(obj.load);
this.player.play(this.volumeVideo);
},
},
mounted() {
const _this = this;
this.player = this.$video(this.$refs.video, this.options, function () {
this.on("volumechange", () => {
// 存储音量
_this.volumeVideo = this.volume();
window.localStorage.volume = this.volume();
});
this.on("play", () => {
this.volume(this.volumeVideo);
});
});
},
};
</script>
<style>
.video-js .vjs-time-control {
display: block !important;
}
.video-js .vjs-remaining-time {
display: none !important;
}
</style>
创作不易,如果这篇文章对你有用,欢迎点赞关注加评论哦
小伙伴们在工作中还遇到过其他应用场景吗,欢迎评论区留言讨论哦。
免责声明:本文由卡卷网编辑并发布,但不代表本站的观点和立场,只提供分享给大家。
- 上一篇:flutter打包apk到底是怎样的才能成功?
- 下一篇:做独立游戏人有多辛苦?
相关推荐
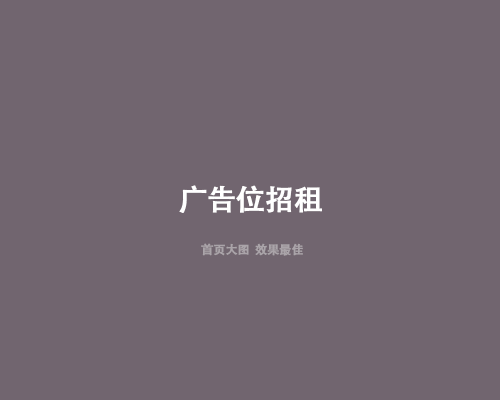
你 发表评论:
欢迎