python程序如何打包为dll,给c#程序调用?
作者:卡卷网发布时间:2024-12-15 00:25浏览数量:128次评论数量:0次
将 Python 程序打包为 DLL 提供给 C# 程序调用,可以通过以下几种方法实现。核心思路是将 Python 代码封装为共享库,供其他语言(如 C#)通过 P/Invoke 或其他方式调用。
方法一:使用 pybind11
或 ctypes
创建共享库
1、准备 Python 代码
将你的 Python 功能封装成一个函数或模块。例如:
# example.py
def add(a, b):
return a + b
2、创建共享库
使用工具将 Python 文件编译为动态链接库(.dll
或 .so
)。
- 安装依赖:
pip install pybind11
- 创建绑定代码
#include <pybind11/pybind11.h>
#include <pybind11/embed.h>
namespace py = pybind11;
int add(int a, int b) {
py::scoped_interpreter guard{}; // 启动 Python 解释器
auto add_func = py::module_::import("example").attr("add");
return add_func(a, b).cast<int>();
}
PYBIND11_MODULE(my_library, m) {
m.def("add", &add, "A function that adds two numbers");
}
- 编译为 DLL: 使用 g++ 或 Visual Studio 编译:
g++ -shared -o my_library.dll -fPIC your_cpp_file.cpp -I<path_to_pybind11> -lpython<version>
3、在 C# 中调用
使用 C# 的 DllImport
调用生成的 DLL:
using System;
using System.Runtime.InteropServices;
class Program {
[DllImport("my_library.dll", EntryPoint = "add")]
public static extern int Add(int a, int b);
static void Main() {
int result = Add(5, 3);
Console.WriteLine($"Result: {result}");
}
}
方法二:使用 cython
生成 DLL
- 安装 Cython
pip install cython
- 编写
.pyx
文件
将 Python 函数声明为可导出的接口:
# example.pyx
cdef public int add(int a, int b):
return a + b
- 生成共享库
使用 Cython 和编译器生成 DLL:
- 创建
setup.py
:python
Copy code
from setuptools import setup
from Cython.Build import cythonize
setup(
ext_modules=cythonize("example.pyx", language_level="3"),
)
- 编译:bash
python setup.py build_ext --inplace
- 将生成的文件重命名为
.dll
(Windows)或.so
(Linux)。
- 在 C# 中调用
和方法一类似,使用DllImport
直接调用生成的 DLL。
方法三:使用 pythonnet
pythonnet
可以在 .NET 应用中直接嵌入 Python 运行时,并通过 Py.Import
调用 Python 模块。具体步骤如下:
- 安装
pythonnet
pip install pythonnet
- 在 C# 中使用 Python
编写 C# 程序直接调用 Python 脚本:
using Python.Runtime;
class Program {
static void Main() {
PythonEngine.Initialize();
using (Py.GIL()) {
dynamic example = Py.Import("example");
int result = example.add(5, 3);
Console.WriteLine($"Result: {result}");
}
PythonEngine.Shutdown();
}
}
优点:无需将 Python 代码转为 DLL,直接调用原生 Python 模块。
缺点:需要在运行时依赖完整的 Python 解释器。
方法四:使用 Boost.Python
或其他工具
如果你的项目复杂,也可以考虑使用其他专用工具,比如 Boost.Python
来生成共享库,这些工具和 pybind11
的用法类似。
对比总结
方法 | 优点 | 缺点 |
---|---|---|
pybind11 | 性能高、支持复杂绑定 | 需要编写 C++ 代码,较复杂 |
cython | 简单、高效,支持静态类型优化 | 不灵活,适合简单接口 |
pythonnet | 无需编译、调用简单 | 依赖完整 Python 运行环境 |
Boost.Python | 强大的 C++ 支持 | 编译复杂,适合高性能要求的场景 |
根据需求和项目复杂度,选择合适的方案即可。
免责声明:本文由卡卷网编辑并发布,但不代表本站的观点和立场,只提供分享给大家。
- 上一篇:博客园为什么要不行了?
- 下一篇:Mac上最好用的软件都有哪些呢?
相关推荐
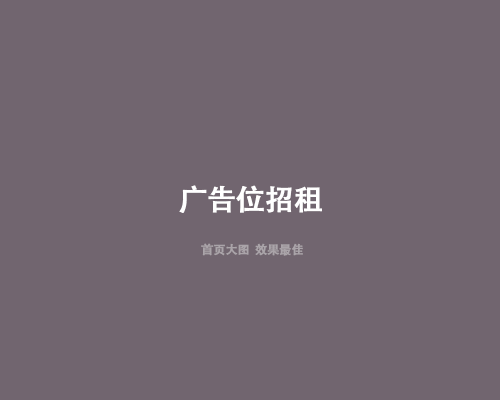
你 发表评论:
欢迎